Light-weight setup of LF console file manager with image, source code and archive previews
Contents
lf, or “list files”, is a single binary file manager, inspired by the ranger file manager, but written in Go. Using this tool, you can navigate really quickly, build up a mental model of the filesystem layout and make modifications with ease.
Out of the box, this is super useful on remote machines or even docker containers where you don’t have access to your normal full configuration, in my case Emacs with dired.
However, you can also have a lot of fun tricking lf out with some extra configuration.
In my case, I wanted a light-weight setup that can preview images (in the terminal!), source code, compressed archives and some other odds and ends, with fast fuzzy file and directory jumping using fzf.
This post shows how to do all of that with the minimum of effort.
Screenshots
First you would probably like to see some examples of what this could look like.
We start with the image previewer:
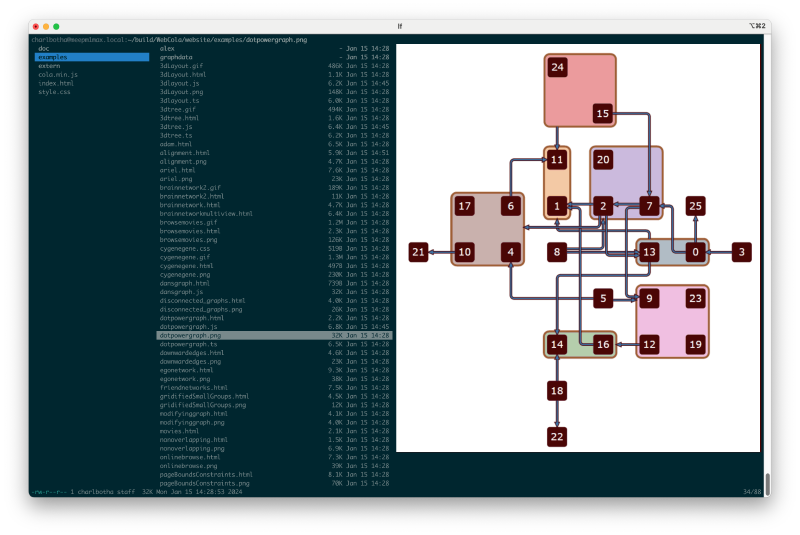
Figure 1: lf previewing an image using chafa and iterm2’s sixel support
Some syntax highlighted source code using the bat previewer with the Solarized (light) theme, which works well on my light and dark mode terminal setup:
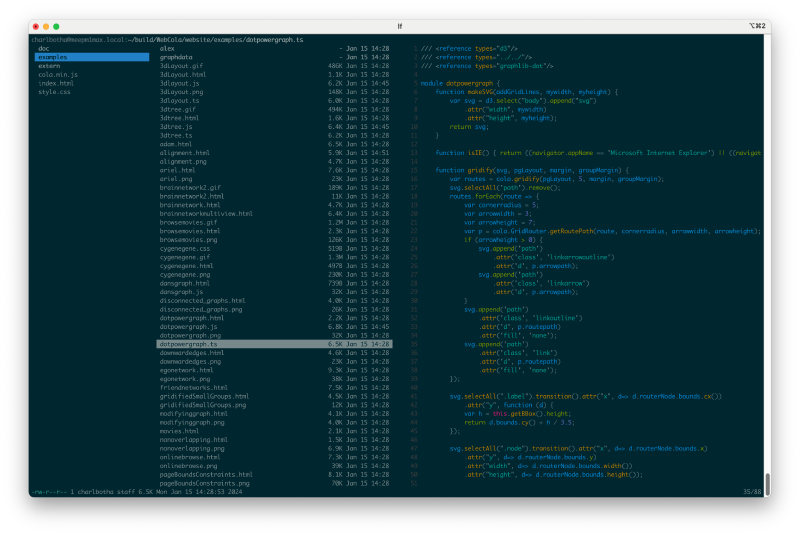
Figure 2: previewing typescript source with bat
Inside the tmux multiplexer, we revert to ASCII rendering, which takes me back:
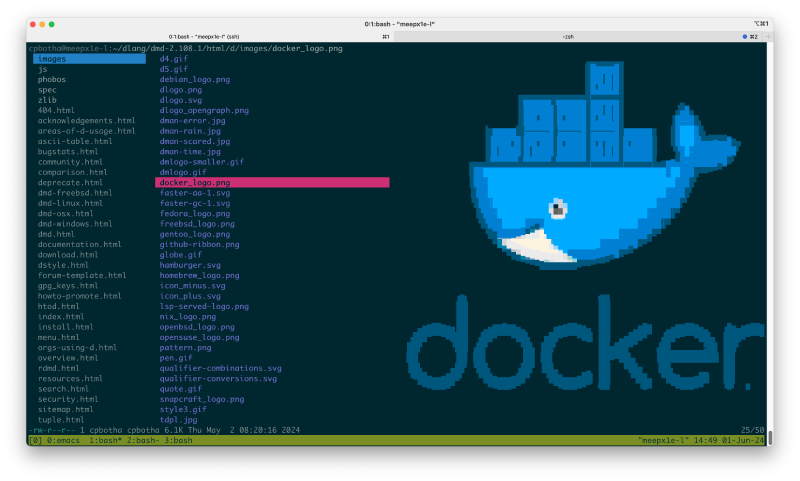
Figure 3: on tmux and windows terminal, we revert to chafa’s amazing ASCII rendering
Finally, listing of archive and package contents using lesspipe:
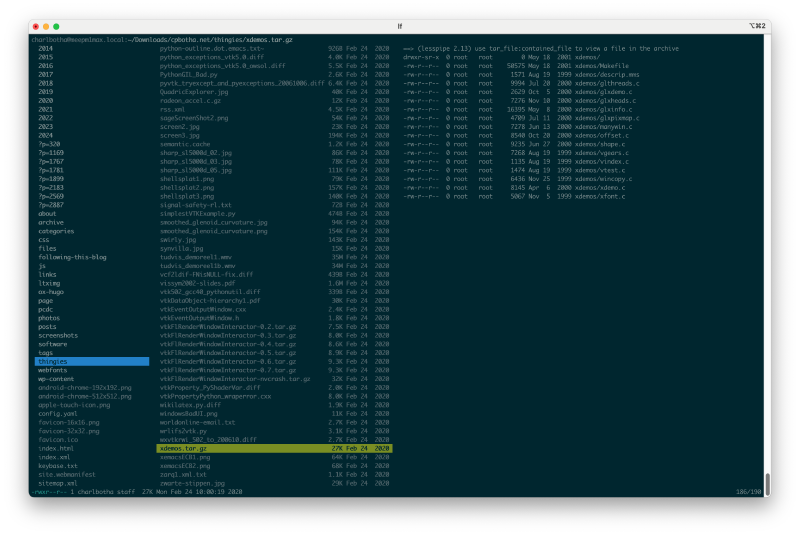
Figure 4: tar.gz contentst using lesspipe, which supports many other formats
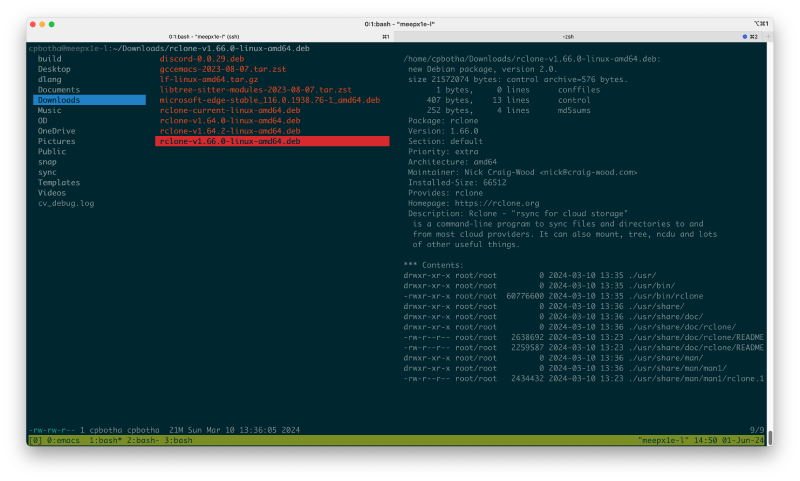
Figure 5: deb package contents and useful metadata
Configuration
This config makes use of a small number of powerful utilities, and hooks them into lf via a preview script:
- file: extract file’s mime type so that the rest of the preview script can decide what to preview it with
- chafa: convert images to sixels or symbols for previewing. Such an amazing and fun project, go look!
- bat: preview many kinds of text files with syntax highlighting
- lesspipe: list contents and metadata of many kinds of archives
- pdftoppm: convert PDFs to images so that they can be previewed via chafa
- command-line fuzzy finder: Invoke with
ctrl-o
for rapidly opening any file or folder nested below your current location
You can install all of these using your package manager (see the preview script below for Debian / Ubuntu and homebrew invocations), after which you must install or configure your lfrc
, preview
and finally your shell configuration.
main lf configuration
Your ~/.config/lfrc
should look as follows.
|
|
As mentioned in the config file, add the following to your ~/.bashrc
or ~/.zshrc
so that when you exit lf
, you won’t be returned to the directory where you started lf
, but rather the one you navigated to.
|
|
preview script
Create the file ~/.config/preview
with the following contents.
Remember to mkdir ~/.cache/lf
for the pdf image caching logic.
|
|