Modify Emacs Deft for recursive directory search
Contents
Update 2014-11-18 I’ve forked the original Deft, added this recursive directory listing feature as well as support for multiple different file extensions, and pushed it all to github as deft-turbo!
Deft is a neat Emacs mode for the Notational Velocity-inspired searching, browsing and editing of a directory of text files. In short, this means that simply start typing, and Deft finds the note that you were looking for. It supports straight text searching and regular expression searching, almost like my own baby nvpy.
When your text files are of a structured type that Emacs supports, such as Markdown, orgmode or something else, you can attain note-taking nirvana.
It looks like this:
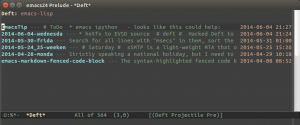
However, I keep my personal text notes in a nested directory structure. I have general notes at the top level, daily journals in their own subdirectory, and projects each in their own subdirectory. Deft unfortunately does not support this out of the box; it only searches in the top-level directory you configure it with.
Fortunately I ran into this bit of example code in the Emacs documentation of all places. After a few small tweaks, it looked like this:
|
|
Note that deft-find-all-files()
takes one argument now, instead of none.
If you copy that function into your deft.el
, replacing the
deft-find-all-files()
that’s already there, and modify
deft-cache-initialize()
so that the first code line reads:
|
|
… your Deft can support nested note directories too!
(I have sent the author this snippet of code (he’s not on github, so no pull request) – we’ll see what happens)